1. [[ Prototype ]]
- 자바스크립트의 객체는 [[Prototype]]라고 하는 숨김 프로퍼티를 갖음
- 이 프로퍼티의 값은 null이거나 다른 객체를 참조
- 다른 객체를 참조하는 경우 참조 대상을 프로토 타입이라 함
- object에서 프로퍼티를 읽으려 할 때 해당 프로퍼티가 없으면 자바스크립트는 자동으로 프로토타입에서 프로퍼티를 찾음(프로토타입 상속)
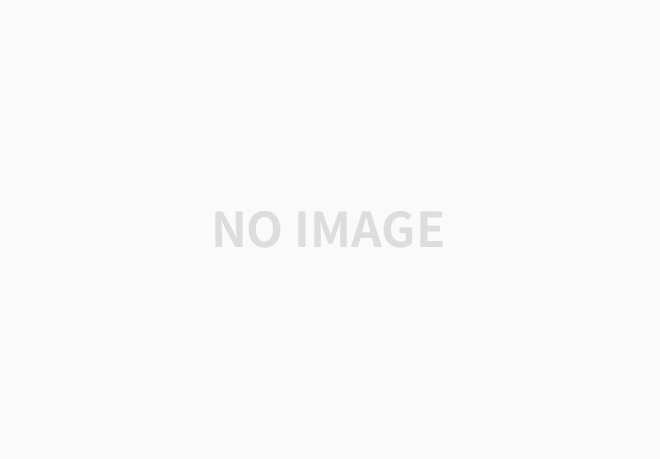
const user = {
activate : true,
login : function(){
console.log('로그인 되었습니다');
}
};
const student = {
passion : true
};
(1) __proto__는 [[Prototype]] 의 getter, setter
student.__proto__=user; 프로퍼타입으로 유저를 참조하도록 해주세요
console.log(student.activate);
=> true
student.login();
=> 로그인 되었습니다
console.log(student.passion);
=> true
student의 프로토타입은 user이다. 또는 student는 user를 상속받는다. 라고 한다
=> 프로토타입에서 상속 받은 프로퍼티를 상속 프로퍼티라고 한다.
(2) 프로토타입 체인
const greedyStudent = {
class : 11,
__proto__ : student //student, user 둘다 참조
};
console.log(greedyStudent.activate);
user에서 상속
console.log(greedyStudent.passion);
student에서 상속
2. prototype feature
const user={
id : 'user',
login : function(){
console.log(`${this.id}님 로그인 되었습니다`);
}
};
const student = {
__proto__ : user
};
(1) student.id = 'user01';
=> user의 id가 변경되지 않고 student 에 id : user01이 추가 됨
(2~3) student.login();
= > user01님 로그인 되었습니다
login 메소드 내의 this는 프로토타입에 영향 받지 않음. 객체의 상태 공유x
(1) 프로토타입은 프로퍼티를 읽을 때만 사용하며 프로퍼티를 추가, 수정, 삭제하는 연산은 객체에 직접 한다.
(2) 메소드 내의 this는 프로토 타입에 영향받지 않으며 메소드를 객체에서 호출했든 프로토타입에서 호출했든 상관없이 this는 언제나 .앞에 있는 객체이다.
(3) 메서드는 공유 되지만 객체의 상태(추가, 수정, 삭제)는 공유되지 않는다
(4)for(let prop in student){
console.log(prop);
(5) let isOwn = student.hasOwnProperty(prop);
if(isOwn){
console.log(`객체 자신의 prop : ${prop}`);
=> 객체 자신의 prop : id
} else {
console.log(`상속 prop : ${prop}`);
=> 상속 prop : login
}
};
(4) for in 반복문은 상속 프로퍼티도 순회 대상에 포함 시킨다
(5) hasOwnProperty(메소드) : key에 대응하는 프로퍼티가 상속 프로퍼티가 아니고, obj에 직접 구현되어 있는 프로퍼티일 때 true 반환한다.
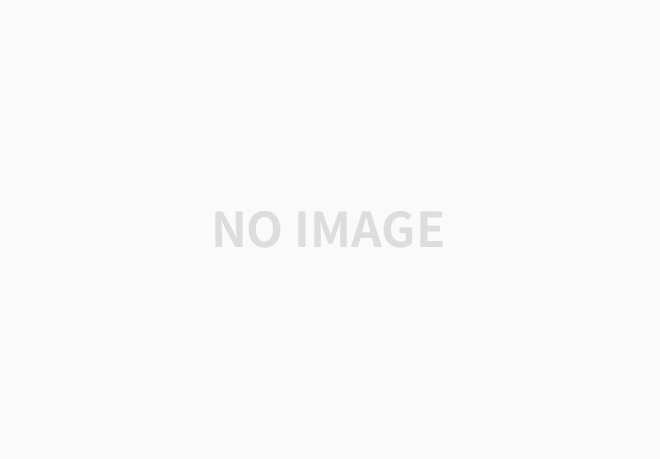
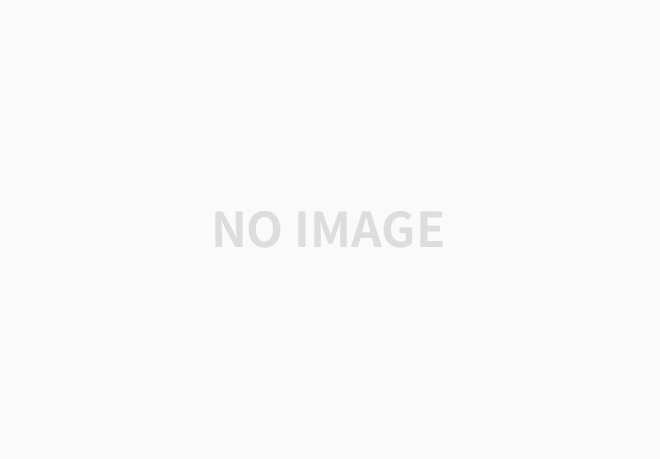
3. function prototype
(1) 생성자 함수 프로토타입
const user = {
activate : true,
login : function(){
console.log(`로그인 되었습니다.`);
}
};
function Student(name){
this.name = name;
}
Student.prototype = user;
=> 프로토타입 속성만들고 = 유저 연결해줌
이때 prototype은 숨김 [[prototype]]이 아닌 일반 프로토타입
let student = new Student("홍길동");
=> F.prototype은 new F를 호출할 때만 사용
생성자 함수쪽에 프로토타입을 설정해두어서
new F를 호출할 때 만들어지는 새로운 객체의 [[Prototype]]을 할당 함.
console.log(student.activate);
=> true
new 연산자를 사용해 만든 객체는 생성자 함수의 프로토타입 정보를 사용해
[[Prototype]]을 설정하는 걸 확인 할 수있다.
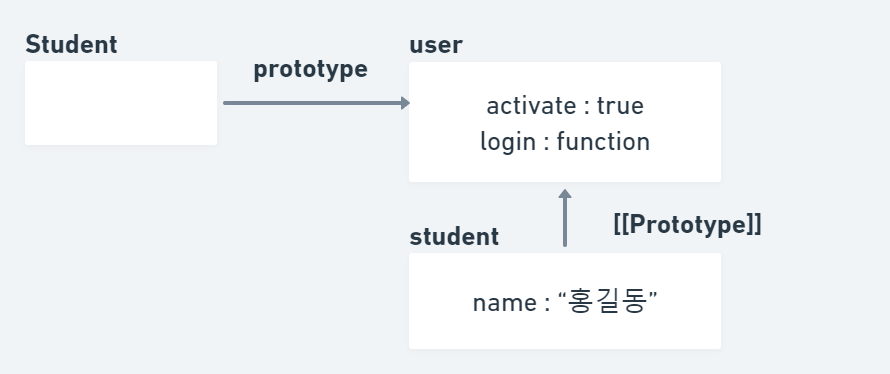
(2) 함수의 디폴트 프로퍼티 프로토타입과 constructor 프로퍼티
- 함수를 만들기만 해도 디폴트 프로퍼티인 prototype이 설정 된다.
- Student.prototype = { constructor : Student };
(자기자신 함수를 생성자로 가지는 기본 객체가 생성됨(상속x 참조값o))
function Student() {}
=> 자기자신 함수를 생성자로 가지는 기본 객체가 생성
let student = new Student(); => { constructor : Student };을 상속 받음
console.log(student.constructor == Student);
=> true
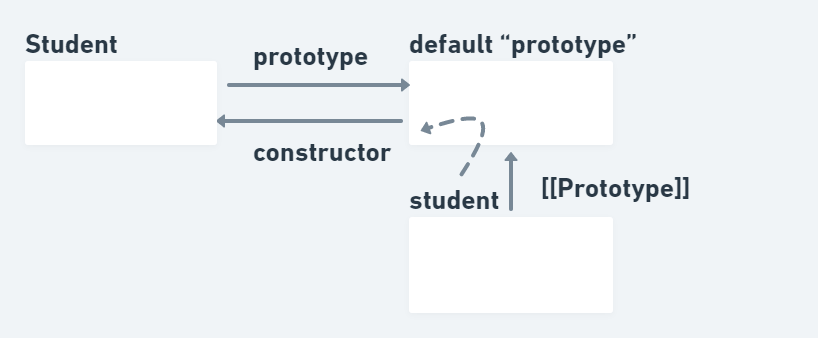
4. object prototype
- Object의 prototype은 toString을 비롯한 다양한 메서드가 구현되어있는 거대한 객체를 참조함.
- new Object()를 호출하거나 리터럴 문법 {...}을 사용해 객체를 만들 때, 새롭게 생성된 객체의 [[Prototype]]은 Object.prototype을 참조
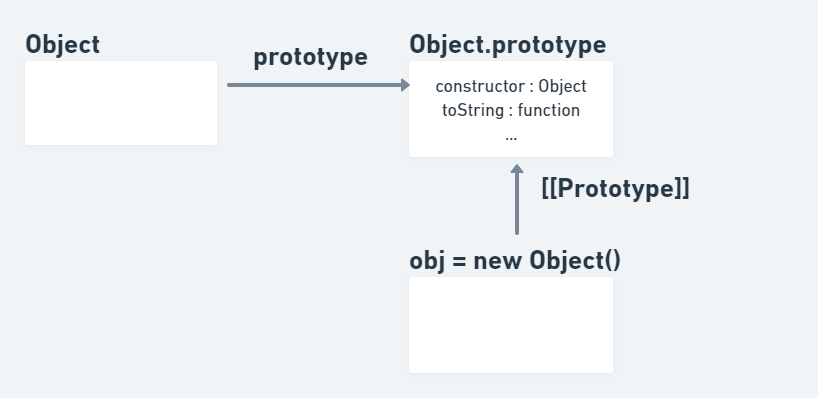
5. built in object prototype
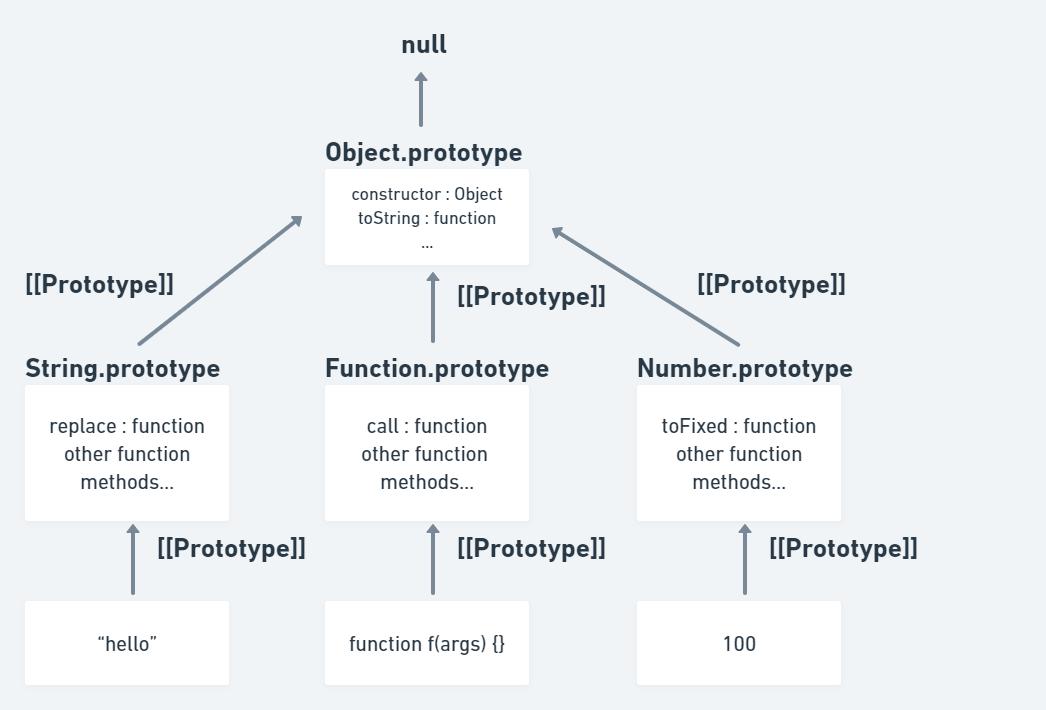
'프로그래밍 > JavaScript' 카테고리의 다른 글
11_자바스크립트 : 배열, 주요메소드 (indexOf , forEach, map, pop...) (0) | 2022.08.04 |
---|---|
09_자바스크립트 : 객체 생성자 함수 (0) | 2022.07.28 |
08_자바스크립트 : scope ( 스코프체인, const, let, 함수 레벨 스코프) (0) | 2022.07.28 |
07_자바스크립트 : 일급 객체 (0) | 2022.07.28 |
06_자바스크립트 : 함수 (화살표함수, 순수함수, 고차함수, 콜백함수, 재귀함수,...) (0) | 2022.07.28 |